Publisher | DevLocker |
---|---|
File size | 157.20kB |
Number of files | 10 |
Latest version | v1.1.0 |
Latest release date | 2024-03-12 01:39:24 |
First release date | 2024-02-19 07:28:15 |
Supported Unity versions | 2018.4.2 or higher |
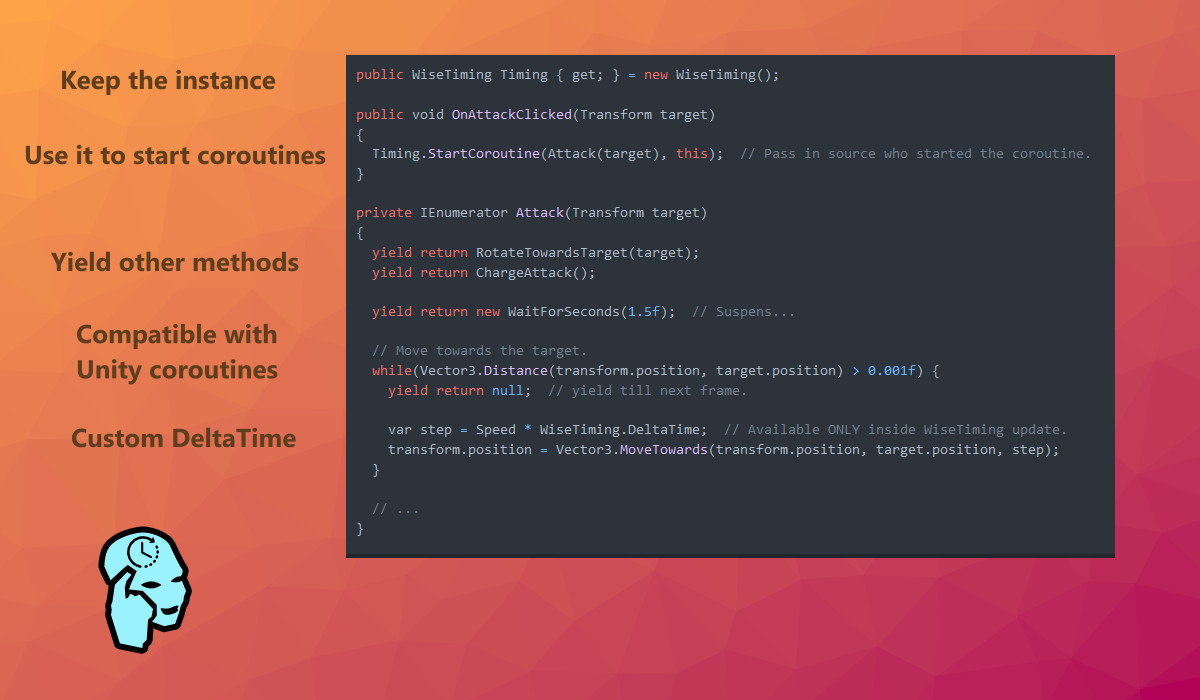
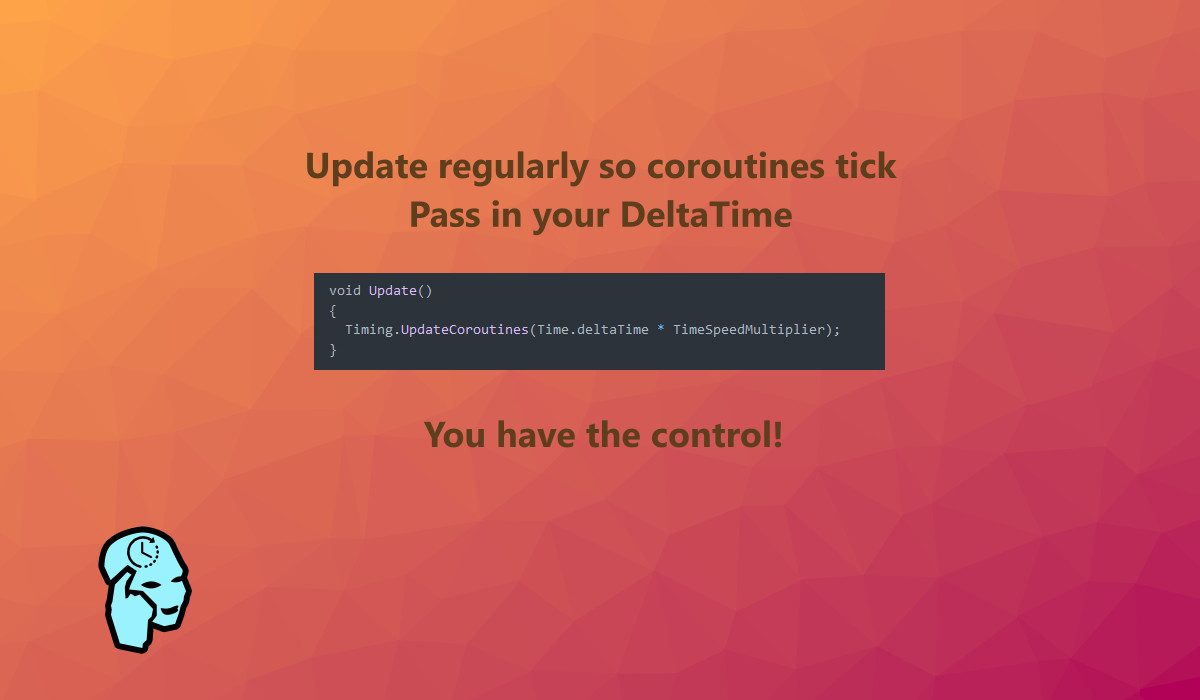
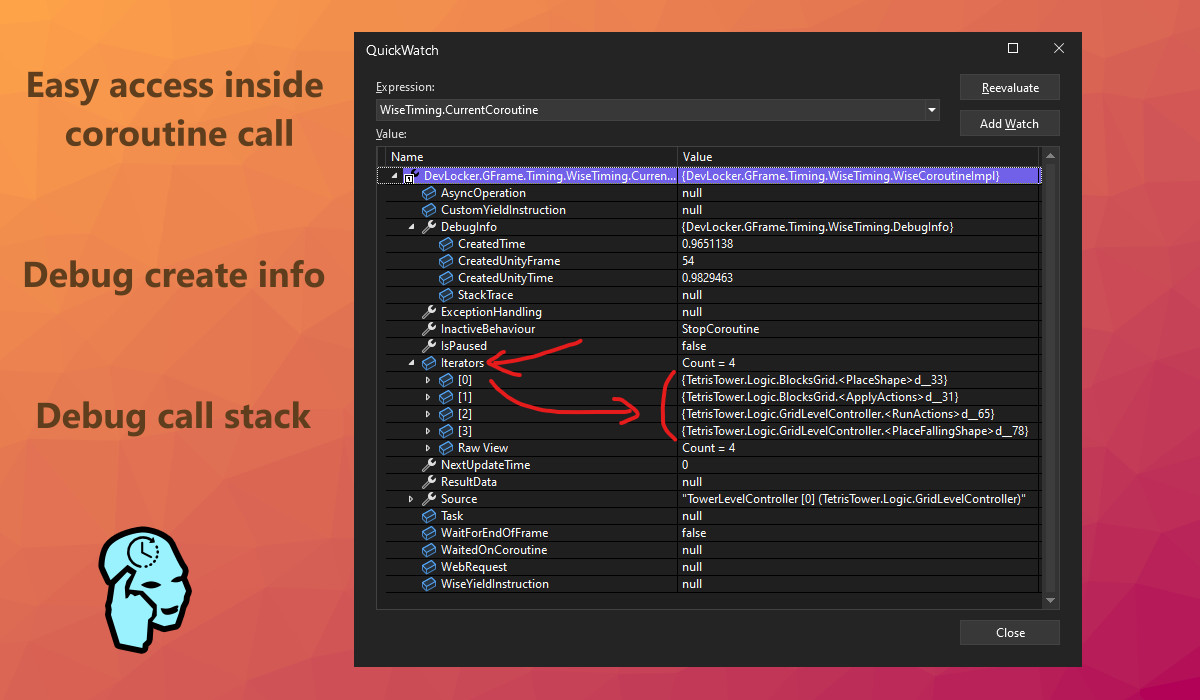
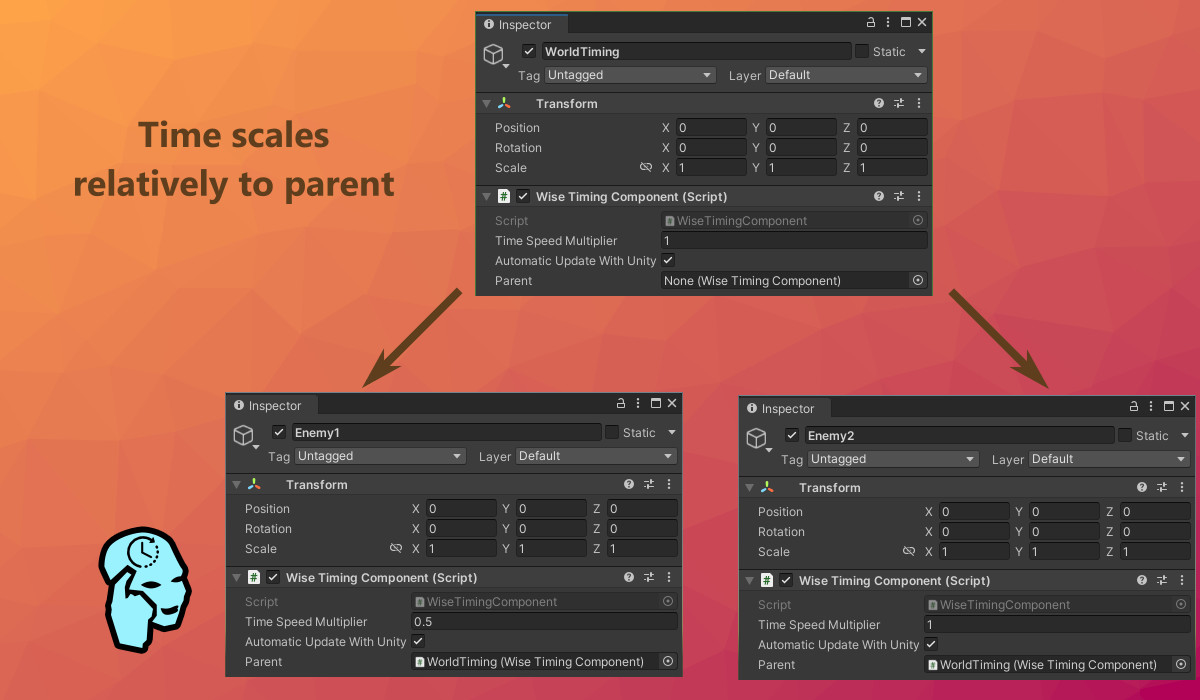
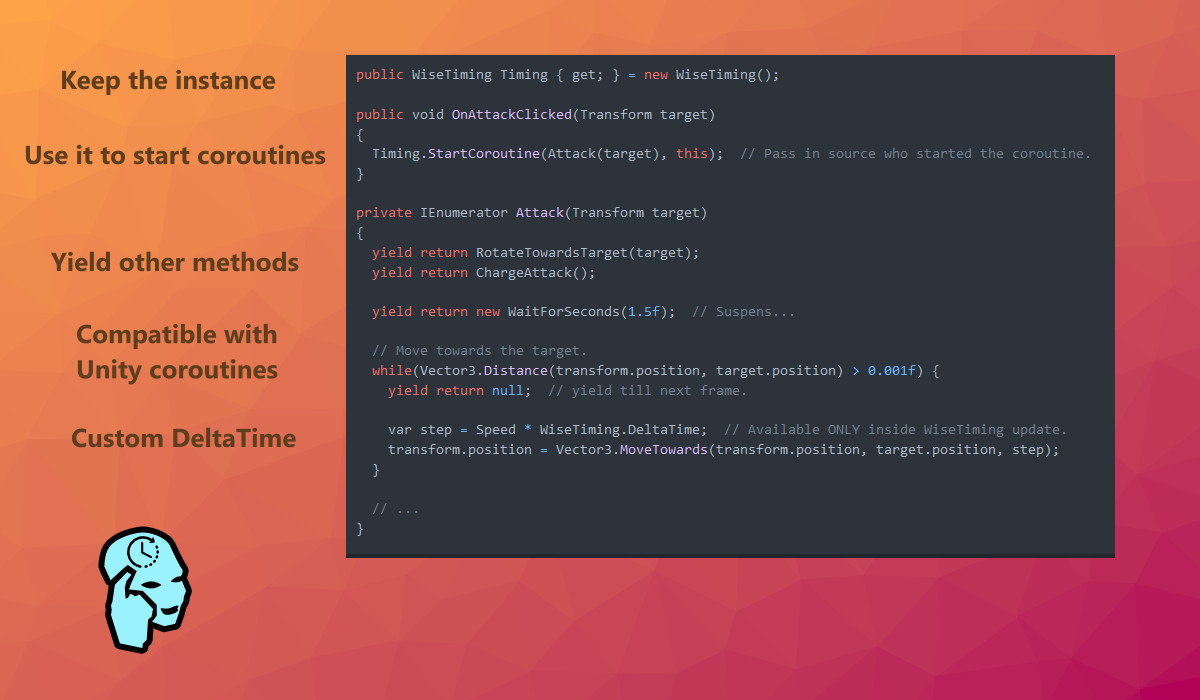
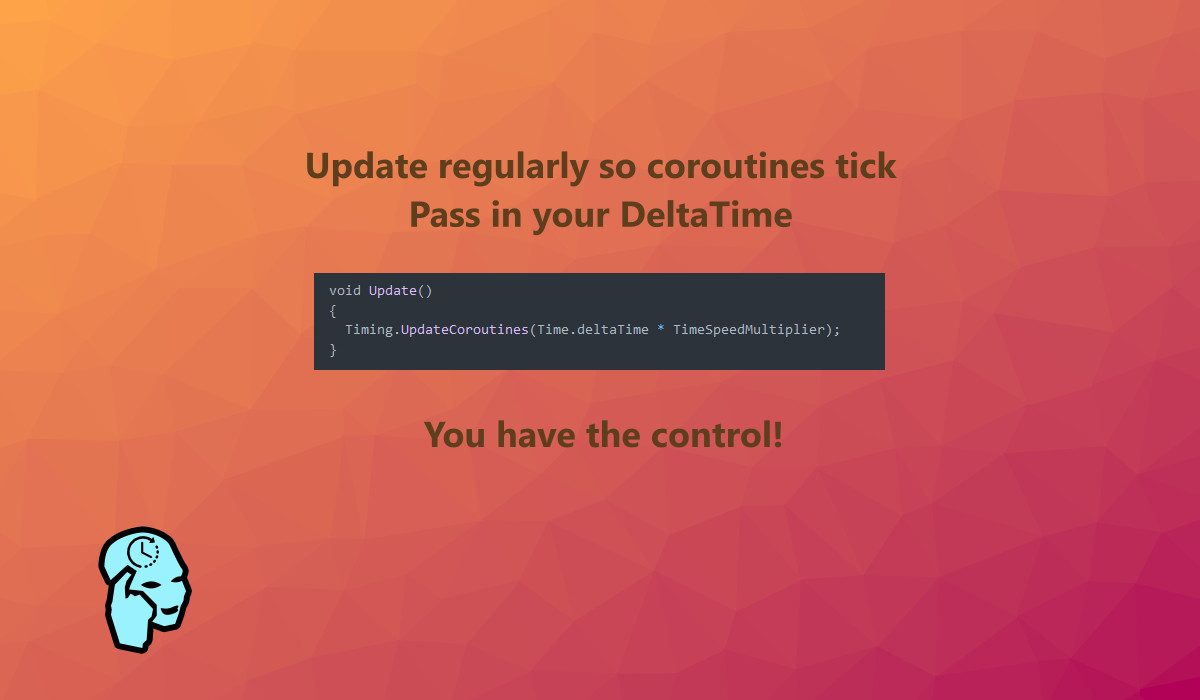
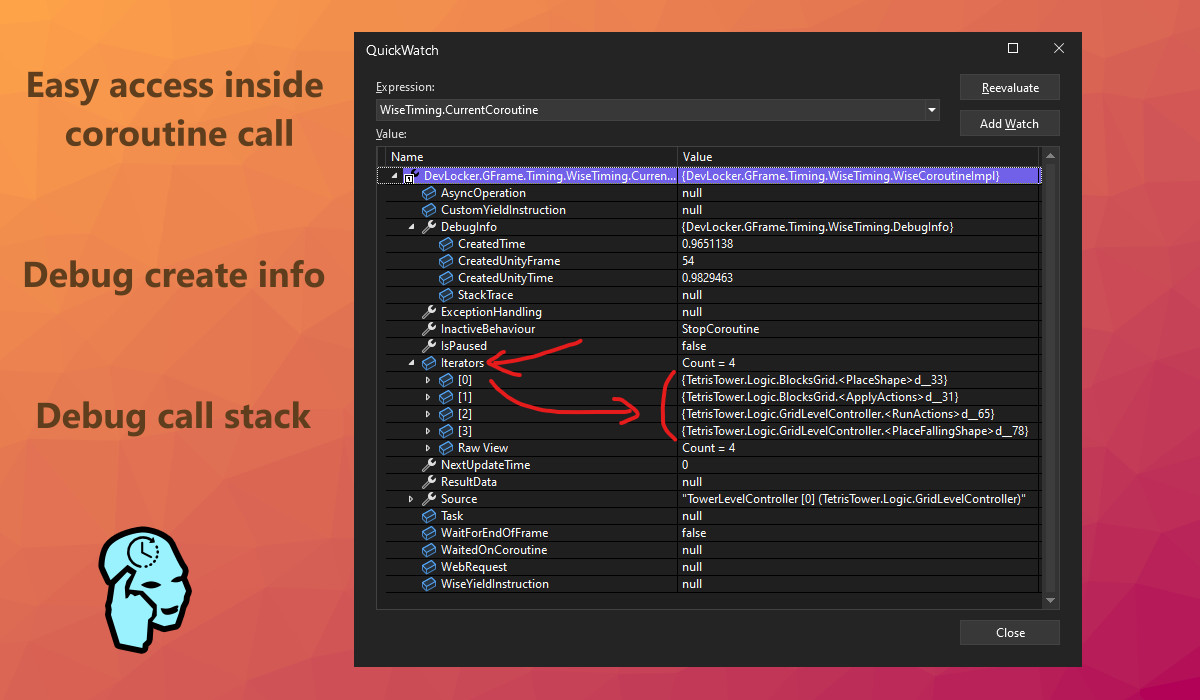
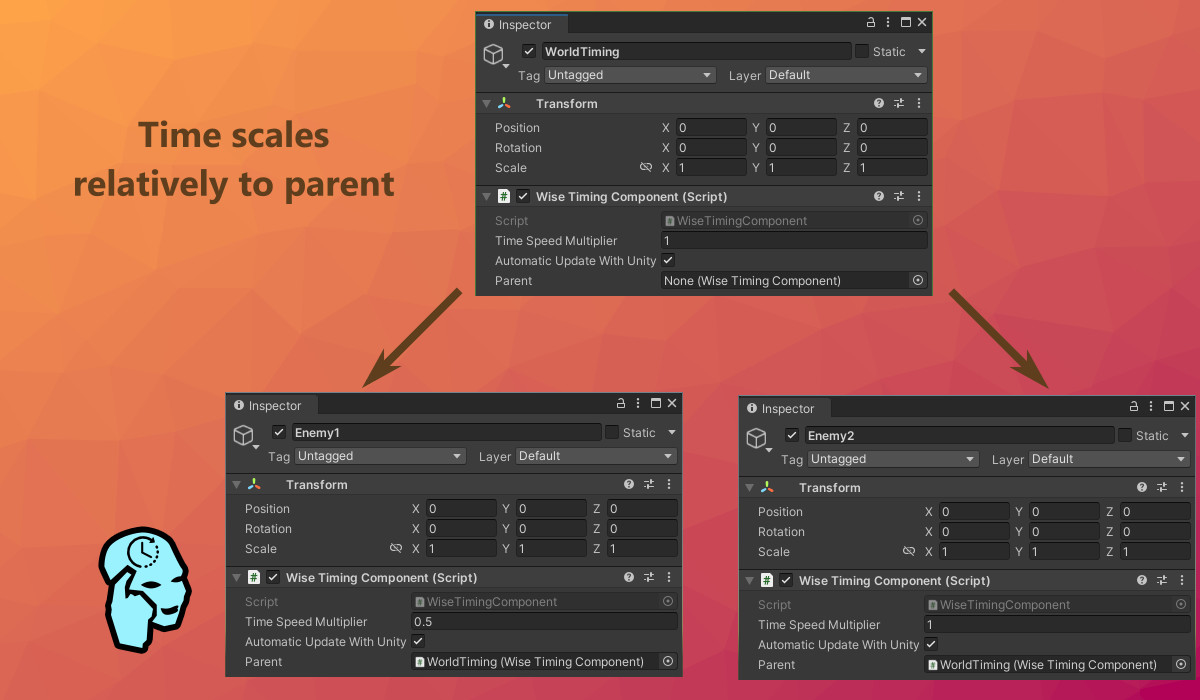
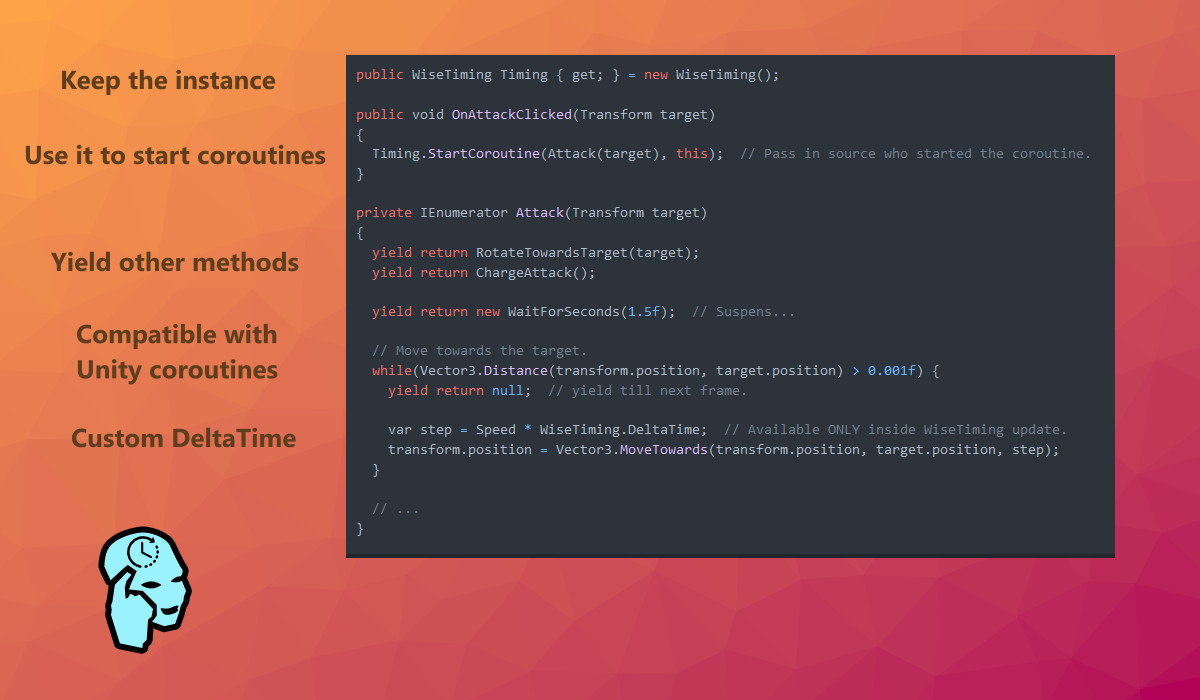
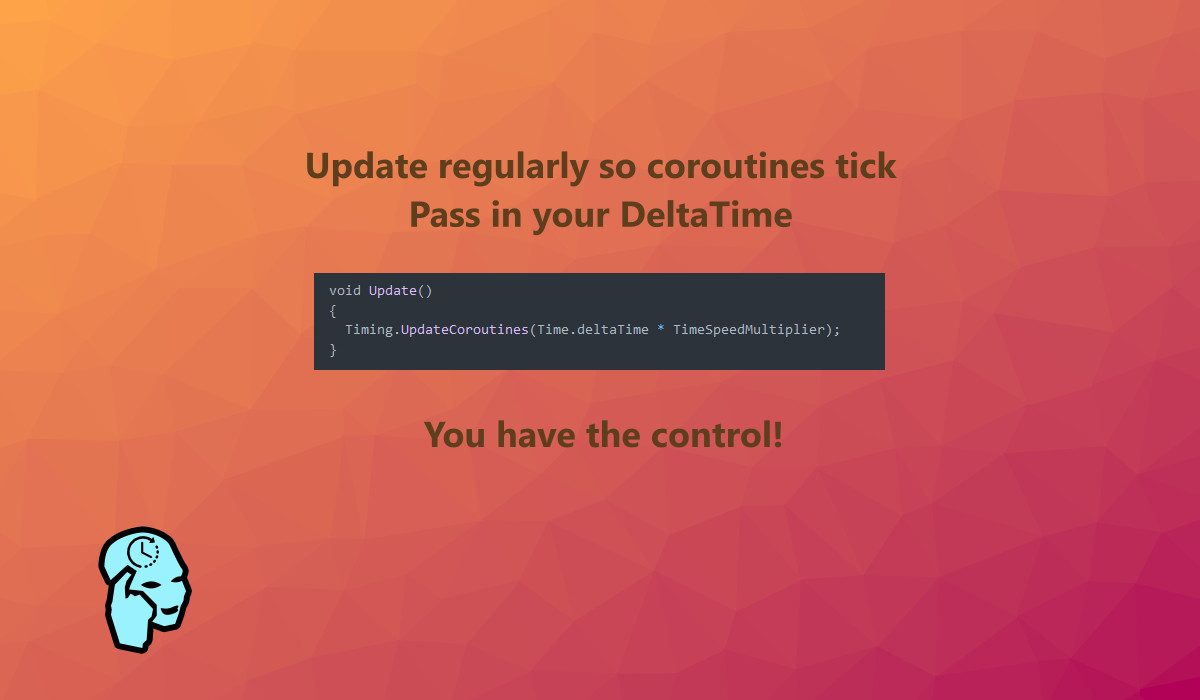
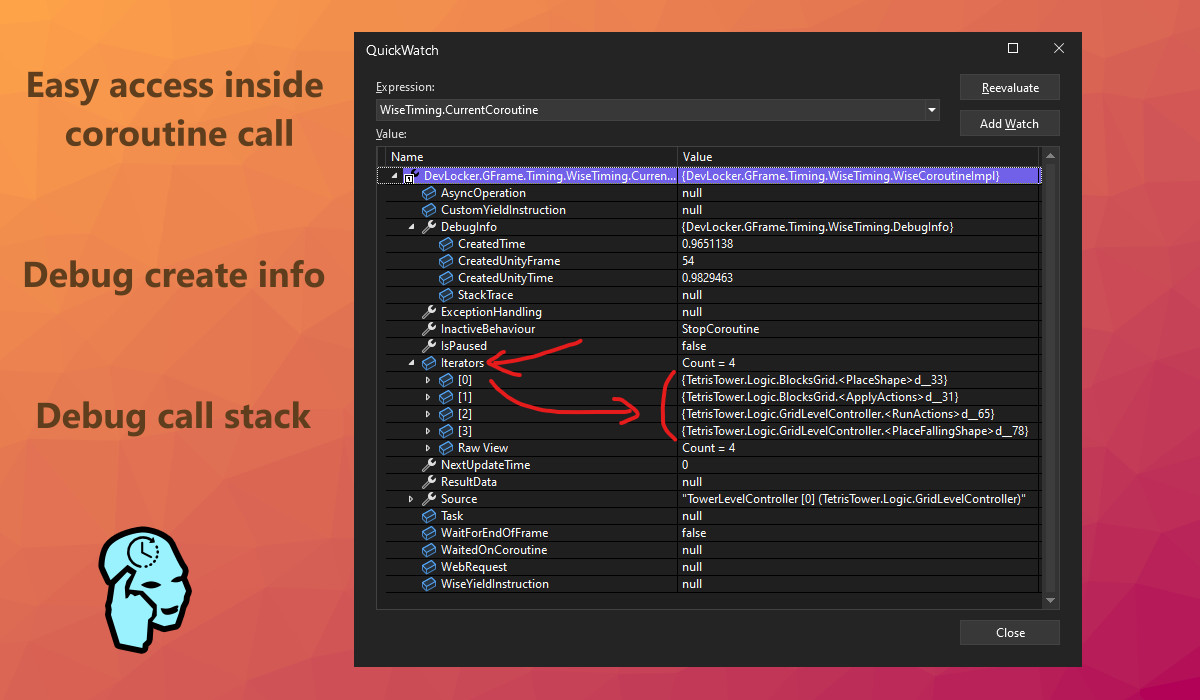
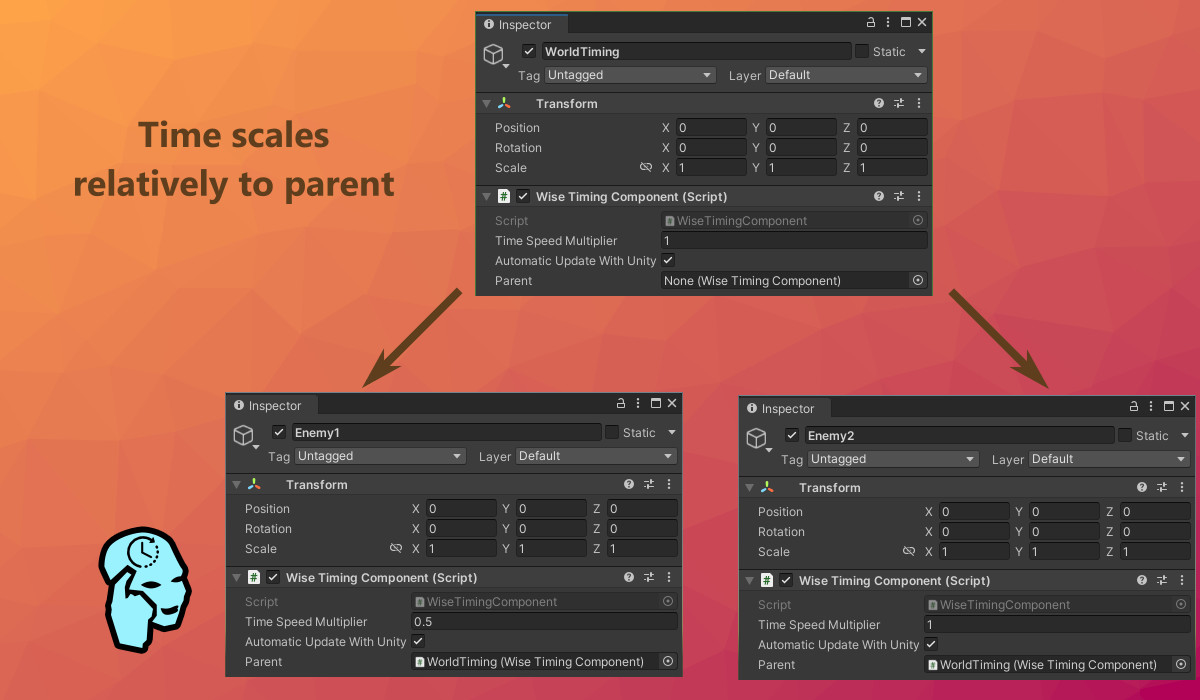
What is this for?
Unity coroutines and Time.deltaTime are the main ways to simulate time in the engine. But they are tied to the Unity life-cycle and frame updates and you have no control over it:
- Time.deltaTime is updated once every frame by the system. You can't feed your own deltaTime values.
- Coroutines are tied to the MonoBehaviour life-cycle - if disabled, coroutines are stopped.
- Coroutines are executed after Update() and before LateUpdate(). The order of their execution is undefined, but it is tied to the MonoBehaviour Update() order (i.e. based on Script Execution Order). This order can often be misleading as it depends on whether the behaviour was created or enabled this frame, where in the hierarchy the object is, etc.
- Can't have multiple time simulations with different deltaTimes (different time speeds).
- Can't manually tick the coroutines when you want.
- Can't iterate or inspect coroutines - there is no API available to do this.
Example usage: imagine that your game has simple logic that doesn't rely on physics (FixedUpdate()). If you want to have a replay functionality, you can just record the input and deltaTime each frame - this will be enough information to reproduce the recorded playthrough. If your code uses Unity coroutines with Time.deltaTime, playback implementation would be impossible as you can't feed Unity your recorded deltaTime.
This is where Wise Timing comes in. Instead of using Unity coroutines and Time.deltaTime, you can use WiseTiming instance. This gives you control over the deltaTime value used by the user code and order of execution. You can also have multiple instances for parallel simulation with different speeds (i.e. each character can have different time speed, slowing down some of them).
Usage
Use WiseTiming the same way you use Unity coroutines, just add in the timing instance and source + use WiseTiming.DeltaTime. For example usage check the code in the screenshot or the github page.
When starting a coroutine, a source must be passed. If source is MonoBehaviour, you can specify if you want the coroutines to stop if the source becomes inactive. If source is destroyed the coroutine is stopped. Source is also used for tracking and debugging.
In order for the coroutines to run, you'll need to call WiseTiming update regularly. Example:
void Update() {
Timing.UpdateCoroutines(Time.deltaTime * TimeSpeedMultiplier);
}
Most used Unity yield instructions are supported: WaitForSeconds, WaitForEndOfFrame, CustomYieldInstruction, UnityWebRequest, AsyncOperation, Task etc. For up-to-date list of supported yield instructions, check the code. WaitForFixedUpdate is not supported because it doesn't make sense.
Read the documentation or the github page for more info.